Corero uses slack to comminicate. Instead of going right to the site people wanted to be able to enter commands like
/who
and
/next this way they could do it within slack and didn't need to go to the webpage everytime they wanted to check.
Slack uses its own form of RESTful commincation between bots and channels inorder to get information. I went through different types
of bots and stuck with the bot that is on the Flask server. The bot is able to communicate with my server and my server to the bot.
When a user types
/next I have told slack to get a post request to my flask server at end point http://myIP
/next.
My server then uses REST API I had developed in my webpage in order to get who is next of shift. I then turn that into a JSON response that
Slack can interpret. Slack then posts the message to a channel that I chose. Below is an exmaple of me using the command in Slack.
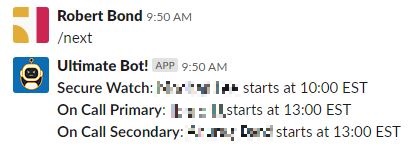
The tougher job for my bot was waiting for an alert to come into the #alert channel and then returning a button with contact information
on the customer. In order to have my bot listen in on people's messages I would have to subscribe it to "events". This way when ever a message
was sent in a channel my server would get a post request from Slack and it would have the text, timestamp, user, channel and more. My server
would then have to check and make sure the user that send the message was an alert. If it was an alert then it will send back a button that
says 'contact'. The button's data would have the server number in it, this is so when it is clicked on I know which customer information to return.
Buttons in Slack are another feature that my server has to respond to. I speficy where Slack shuold send a post request when the button is clicked on.
From there I can get which button was clicked on and then can look up the customer information. To lookup customer info I use Splunk REST API and
then parse the information I need out of the look-up file. A few months later I updated my bot to work differently. I was told "people want to see
a few of the contact fields everytime" and "it's annoying having to scroll down the chat when clicking on a button farther back". To fix this I
have my server do a Splunk look-up before sending the button. This way it can send a few fields next to the 'contact' button.

In order to have my bot 'edit' my message and add more info instead of making a new one I would need two parameters. The timestamp of the message
I want to edit and the text I want to change it to. Getting the timestamp and saving it was the hard part.
I wouldn't know the exact timestamp unless slack tells me. When ever a message is sent in our wrokspace my bot gets the post request from Slack because
every message is an 'event'. My server fixes this by saving the timestamp and text of every message that my bot sends along with assigning the buttons lookup ids
that match with its saved info.
- Alert comes in to #alert channel
- Server sees a message was send with an alert
- Bot sends buttons with random UNIQUE id (326457 for an example)
- Server sees a message was sent by itself with buttons
- Server writes the following to a file
- Button ID (326457 for an example)
- Time Stamp of message
- Text that was in message
- User clicks the button
- Server gets post request from Slack with the button id
- Server reads file to find id
- Server saves the time stamp and original text to variables
- Server removes id from file (button won't be used again)
- Knowing the time stamp and text it can update the message
At first I had my server saving the ids and such to a dictionary. The problem with this is that any variable in a script is volatile. To get around this
I learned about writting, reading and appending to files in Python. This way when I needed to reboot the server I would not lose all of the button ids. I
also would not have to worry about my server running out of memory.